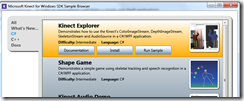
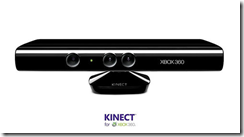
I have the opportunity to borrow a Kinect hardware – to make an application( I will make a simple anti-theft application).
The steps for developing in Kinect are:
- Download the SDK from http://www.microsoft.com/en-us/kinectforwindows/develop/overview.aspx – it contains the drivers also
- Connect Kinect to USB , plug in the socket
- Run the Kinect Explorer or Kinect Shape Game from Kinect SDK Sample Browser installed at 1. Ensure it works.
- Read the Kinect Explorer source –it is SO clear!
- Read documentation – finally, you should RTFM

Optional resources:
- http://kinectcontrib.codeplex.com/ – Visual Studio template for Skeleton, Audio, Video. Simple example that works ( simpler than Kinect Explorer !)
- http://c4fkinect.codeplex.com/ – added methods to Kinect.
- http://kinecttoolbox.codeplex.com/ – detection of gesture.
Example 1: Integrating saving image in Kinect Explorer when a skeleton is detected
Add reference to optional resource 2( either download , either via Nuget)
Search for KinectAllFramesReady in KinectSkeletonViewer.xaml.cs and put this code
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | var takePic = this .skeletonData.Count(item => item.TrackingState == SkeletonTrackingState.Tracked) > 0;
if (takePic)
{
DateTime imgDate = DateTime.Now;
string imageName = "andrei" + imgDate.ToString( "yyyyMMdd_HHmmss" ) + ".jpg" ;
if (!File.Exists(imageName))
{
using ( var image = e.OpenColorImageFrame())
{
if (image != null && this .skeletonData.Length > 0 && this .skeletonData.Count(item => item.TrackingState != SkeletonTrackingState.NotTracked) > 0)
if (image != null && takePic)
{
var x = image.ToBitmapSource();
var b = Save(x, ImageFormat.Jpeg);
var t = Task.Factory.StartNew(
(img) =>
{
imgDate = DateTime.Now;
imageName = "andrei" + imgDate.ToString( "yyyyMMdd_HHmmss" ) + ".jpg" ;
if (File.Exists(imageName))
return ;
byte [] i = img as byte [];
if (i != null )
{
File.WriteAllBytes(imageName, i);
};
}, b);
}
}
}
}
|
Example 2 : Detecting circle by right hand in Kinect Explorer when a skeleton is detected
Add reference to optional resource 3( either download , either via Nuget)
Download circleKB.save file and put in your project. Ensure “Copy to output directory” is “copy always/copy if newer”
Add a variable named
1 | TemplatedGestureDetector circleGestureRecognizer;
|
In KinectSkeletonViewer.xaml.cs in constructor put
1 2 3 4 5 6 | using (Stream recordStream = File.Open( "circleKB.save" , FileMode.Open))
{
circleGestureRecognizer = new TemplatedGestureDetector( "Circle" , recordStream);
circleGestureRecognizer.OnGestureDetected += new Action< string >(circleGestureRecognizer_OnGestureDetected);
}
|
Search for KinectAllFramesReady in KinectSkeletonViewer.xaml.cs and put this code
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 | foreach (Joint joint in skeleton.Joints)
{
Point mappedPoint = this .GetPosition2DLocation(depthImageFrame, joint.Position);
jointMapping[joint.JointType] = new JointMapping
{
Joint = joint,
MappedPoint = mappedPoint
};
if ((joint.TrackingState == JointTrackingState.Tracked) && (joint.JointType == JointType.HandRight))
{
circleGestureRecognizer.Add(joint.Position, Kinect);
}
}
|
That will be all…
If you have developed with Kinect, please share your sources as comments.