Saving and retrieving application settings
Every application that I have made needs at minimum two things, that we will put on the generic term “ settings “:
1. “remember” their state – information that are read at the beginning, write at the end of the program . We will name those “local settings” because there are different by each user that uses the application.
2. Connect with other applications (databases, web services, other programs ) and remember the “connection” settings – information that is read and does not change often ( number of writes is minimized). We will name this “global settings”.Most of the time the writing is realized by setup and/or a new application dedicated to application administration.
Usually the settings are saved in a file persisted on local hard disk. From the programmer perspective, there are four questions that arise:
1. when to save
2. where to save
3. how to save
4. how to make the information confidential.
When to save
The saving can be done in two separate moments: on installing the application and in running the application. Usually, the global settings(information that does not change often such as database connection) is persisted on application setup and read when the application starts. The local settings information (such as last action of the user) is read when the application starts and it is write at the application end.
Where to save
For a desktop application there are two places to save the data for a application : the folder where the applications is installed by the user (usually %programfiles% , can be obtained in .NET with Path.GetDirectoryName( Assembly.GetEntryAssembly().GetName().CodeBase)) and the local folder for application data for this user (%USERPROFILE%\AppData\Local – obtained in .NET with
Environment.GetFolderPath(Environment.SpecialFolder.LocalApplicationData)
). The first one is for global settings, the other one for local user settings.
How to save
We will enumerate here several methods, remaining for you the task to choose between the best. We will deliberately skip the method in which you use a file and you wrote information there in a proprietary format.
In the next examples we will save/retrieve two named properties : DatabaseConnection and LastAction
Save method 1 : from Settings within Visual Studio
You can create settings for your project very easy. Load your project in Visual Studio, click on toolbar Project=>Properties, select the tab “Settings” and create the settings. In the picture you will see two settings, one with user scope and one with application scope:
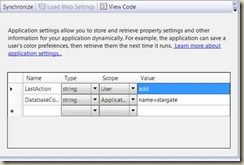
By default Visual Studio creates a class named “Settings” and puts code for retrieving/storing the settings that you create. More – the application settings does not have a “set” by default – it is normal since it is stored in application config file – an ordinary user cannot write this setting.
The code for retrieving/saving the settings is the following:
Console.WriteLine(Properties.Settings.Default.DatabaseConnection);
Console.WriteLine(Properties.Settings.Default.LastAction);
Properties.Settings.Default.LastAction = “difference”;
Properties.Settings.Default.Save();
//you can reset the values by
//Properties.Settings.Default.Reset();
For more details please refer to the following links :
· Application Settings Architecture , http://msdn.microsoft.com/en-us/library/8eyb2ct1.aspx
· Application Settings Overview , http://msdn.microsoft.com/en-us/library/k4s6c3a0.aspx
Video tutorial : http://www.youtube.com/watch?v=-JDoZU0HBBo&feature=PlayList&p=70009BDDADB00AEF&index=1
Save method 2 : from application configuration file
You can read/save directly from application configuration file. Add an application configuration file to the project and a reference to System.Configuration . In the app.config file add
<appSettings>
<add key=”DatabaseConnection” value=”name=Stargate”/>
<add key=”LastAction” value=”add”/>
</appSettings>
And the code is the following:
var app=ConfigurationManager.AppSettings;
foreach (var key in app.AllKeys)
{
Console.WriteLine(string.Format(“{0}={1}”,key,app[key]));
}
Observation 1 : Please ensure you put in the app.config file file just global settings. Otherwise the application must have administrative rights to modify the app.config file.
Observation 2 : For asp.net applications , the modifying of Web.config will result in restarting the application – that is NOT a good behaviour.
Video Tutorial : http://www.youtube.com/watch?v=Axkt_KE0JX4&feature=PlayList&p=70009BDDADB00AEF&index=2
Save method 3 , create a new configuration :
This method is a combinations between method 2 and 3 : we have an intermediate class to save and we save in the config file . It is somewhat more to write about, but it is more safe – and can be modified and/or tested easily.
First we create the class :
public class MyConfig : ConfigurationSection
{
[ConfigurationProperty(“DatabaseConnection”)]
public string DatabaseConnection
{
get
{
return base[“DatabaseConnection”].ToString();
}
set
{
base[“DatabaseConnection”] = value;
}
}
[ConfigurationProperty(“LastAction”)]
public string LastAction
{
get
{
return base[“LastAction”].ToString();
}
set
{
base[“LastAction”] = value;
}
}
}
Next , we put in the application configuration file the settings :
<configSections>
<sectionGroup name=”MyApplicationSettings”>
<section name=”MyConfig” type=”AppSettingsConsole.MyConfig, AppSettingsConsole” restartOnExternalChanges=”false” allowDefinition=”Everywhere” />
</sectionGroup>
</configSections>
<MyApplicationSettings>
<MyConfig DatabaseConnection=”name=Stargate” LastAction=”add”>
</MyConfig>
</MyApplicationSettings>
And third we wrote code to retrieve :
MyConfig m = (MyConfig)System.Configuration.ConfigurationManager.GetSection(“MyApplicationSettings/MyConfig”);
Console.WriteLine(m.DatabaseConnection);
Console.WriteLine(m.LastAction);
Video Tutorial : http://www.youtube.com/watch?v=oglDeV7sc94&feature=PlayList&p=70009BDDADB00AEF&index=3
Save method 4, XML file :
This is the method with the more liberty. We can define any class we want to preserve properties – we just need to serialize the class.
public class MySettings
{
static XmlSerializer xs;
static MySettings()
{
xs = new XmlSerializer(typeof(MySettings));
}
public string DatabaseConnection { get; set; }
public string LastAction { get; set; }
public void SaveToFile(string NameFile)
{
using (StreamWriter sw = new StreamWriter(NameFile))
{
xs.Serialize(sw, this);
}
}
public static MySettings RetrieveFromFile(string NameFile)
{
using (StreamReader sw = new StreamReader(NameFile))
{
return xs.Deserialize(sw) as MySettings;
}
}
}
And the code to save is easier :
string folderPath = Environment.GetFolderPath(Environment.SpecialFolder.LocalApplicationData);
string FileName = Path.Combine(folderPath, “mysettings.txt”);
MySettings ms = new MySettings();
ms.DatabaseConnection = “name=Stargate”;
ms.LastAction = “add”;
ms.SaveToFile(FileName);
MySettings msRetrieve = MySettings.RetrieveFromFile(FileName);
Console.WriteLine(ms.LastAction + ” — ” + ms.DatabaseConnection);
Video Tutorial : http://www.youtube.com/watch?v=MtK3zZYjfS0&feature=PlayList&p=70009BDDADB00AEF&index=0
Save method 5, Ini Files
http://jachman.wordpress.com/2006/09/11/how-to-access-ini-files-in-c-net/
http://www.codeproject.com/KB/cross-platform/INIFile.aspx
Save method 6, Database
It’s obvious that you can have a table with 3 columns :Object, Name and Value. Access this column with EF, L2S , NHibernate or any other framework
Save method 7, Registry
Just the code
using (RegistryKey r = Registry.CurrentUser.CreateSubKey(@”Software\App”))
{
{
r.SetValue(“myvalue”, “1”, RegistryValueKind.String);
}
}
using (RegistryKey r = Registry.CurrentUser.CreateSubKey(@”Software\App”))
{
Console.WriteLine(r.GetValue(“myvalue”));
}
Video Tutorial : http://www.youtube.com/watch?v=UeBPTbWgthM&feature=PlayList&p=70009BDDADB00AEF&index=4
Summary
In this chapter we have shown different ways to store application settings in various file formats. A final recommendation is to store application global data in config file (either web.config, either app.config) and local user in XML file ( in
Environment.GetFolderPath(Environment.SpecialFolder.LocalApplicationData)
folder). More, for each desktop application please provide a way to edit the config file – and make sure you request elevated privileges.